So with my MongoDB server running it was time to write some code against it to create collections, add documents to it, update, delete etc
Downloaded the C# driver, fired up VS 2010 and wrote some code
A simple winform app which on load connected to the db “test” and got the collection “books” and then buttons to create “documents” (one at a time and in a batch), read “documents” (top one and all), dropping all documents, updating based on a condition and removing one document from a collection.
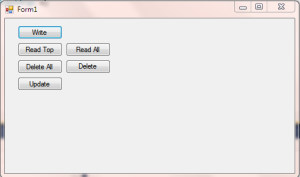
Here is the code…
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using MongoDB.Bson;
using MongoDB.Driver;
namespace FirstMongoApp
{
public partial class Form1 : Form
{
MongoDatabase database;
MongoCollection<BsonDocument> books = null;
public Form1()
{
InitializeComponent();
}
//Write records to a table…errr Collection!!!
private void button1_Click(object sender, EventArgs e)
{
books = database.GetCollection<BsonDocument>(“books”);
BsonDocument book = new BsonDocument {
{ “author”, “Ernest Hemingway” },
{ “title”, “For Whom the Bell Tolls” },
{ “rating”, “5.1” }
};
books.Insert(book);
book = new BsonDocument {
{ “author”, “Bad Hemingway” },
{ “title”, “Bad Bell Tolls” },
{ “price”, “9.99” }
};
books.Insert(book);
BsonDocument[] batch = {
new BsonDocument {
{ “author”, “Kurt Vonnegut” },
{ “title”, “Cat’s Cradle” }
},
new BsonDocument {
{ “author”, “Kurt Vonnegut” },
{ “title”, “Slaughterhouse-Five” }
}
};
books.InsertBatch(batch);
}
//Initialize
private void Form1_Load(object sender, EventArgs e)
{
var connectionString = “mongodb://localhost”;
var client = new MongoClient(connectionString);
var server = client.GetServer();
database= server.GetDatabase(“test”); // WriteConcern defaulted to Acknowledged
books = database.GetCollection<BsonDocument>(“books”);
}
//Read one
private void button2_Click(object sender, EventArgs e)
{
BsonDocument doc = books.FindOne();
if (!(doc == null))
{
foreach (BsonElement element in doc.Elements)
{
MessageBox.Show(element.Name + ” ” + element.Value);
}
}
}
//Read all
private void button4_Click(object sender, EventArgs e)
{
if (!(books == null))
{
var docs = books.FindAll();
foreach (var book in docs)
{
foreach (BsonElement element in book.Elements)
{
MessageBox.Show(element.Name + ” ” + element.Value);
}
}
}
}
//Delete al
private void button3_Click(object sender, EventArgs e)
{
if (!(books == null))
{
books.Drop();
}
}
//Update
private void button5_Click(object sender, EventArgs e)
{
var query = new QueryDocument{
{ “author”, “Kurt Vonnegut” }
};
var update = new UpdateDocument {
{ “$set”, new BsonDocument(“title”, “Cat’s Kradle”) }
};
books.Update(query, update);
query = new QueryDocument{
{ “author”, “Bad Hemingway” }
};
update = new UpdateDocument {
{ “$set”, new BsonDocument(“title”, “Cat’s Kradle”) }
};
books.Update(query, update);
}
//Delete
private void button6_Click(object sender, EventArgs e)
{
var query = new QueryDocument{
{ “author”, “Bad Hemingway” }
};
books.Remove(query);
}
}
}
ok so now where do i use this in real life !!!